[udemy] Python 부트캠프 : 100개의 프로젝트로 Python 개발 완전 정복을 통해 학습하고 있습니다.
파이썬 함수(Function) 생성하기
- def를 통해 원하는 함수를 만들어서 사용할 수 있음.
def my_function():
# Do this
# Then do this
# Finally do this
- 첫 번째는 실제로 해당 함수가 무엇을 해야하는지 지정(Defining)하기 위해 def 키워드를 사용하고 함수에 이름을 지정하고, 그 다음에 괄호와 콜론을 사용한 다음 함수에 포함될 코드 줄을 작성함.
해당 함수가 호출될 때 실행될 함수에 들어가는 코드 줄은 들여쓰기가 되어야 함.
- 두 번째는 해당 함수를 사용하는 것인데, 프로그래밍 용어로는 함수를 호출(Calling)한다고 함.
함수를 호출하려면 이름과 괄호를 지정하면 되고, 컴퓨터는 해당 코드를 보고 함수 내부에 정의된 모든 내용을 실행해야 한다는 것을 알고 실행함.
❓ 아래 사이트에서 로봇이 움직이게 하는 게임 과제 실행하기
Q. 해당 로봇이 깃발 있는 지점까지 도달할 수 있도록 코드 만들기
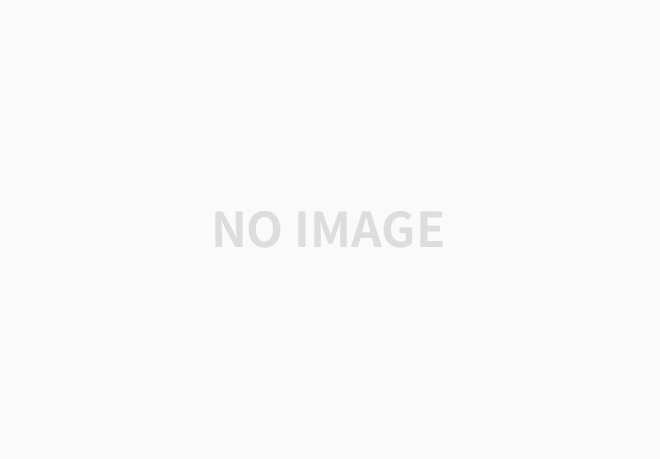
def turn_right():
turn_left()
turn_left()
turn_left()
def jump():
move()
turn_left()
move()
turn_right()
move()
turn_right()
move()
turn_left()
for go in range(1, 7):
jump()
→ def로 오른쪽으로 도는 함수를 만들고 하나의 블록을 점프하기 위한 코드를 jump라는 함수로 만들어 묶어준 뒤 for문을 사용하여 6번 반복하도록 만듦.
파이썬 들여쓰기(Indentation)
- 들여쓰기란 오른쪽으로 네 칸 들어간 것을 의미함.
* 옛날부터 개발자들 사이에서 tab키를 사용하는지 스페이스 네번을 누르는지 분쟁이 있었다고 함
파이썬에서는 tab이 아닌 스페이스 네번을 눌러 공백을 만들어야 한다고 함.
근데 탭과 스페이스를 같이 사용할 수는 없음.
그리고 대부분의 코드 편집기에서 공백을 설정할 수 있다고 함.
(https://peps.python.org/pep-0008/#indentation, https://peps.python.org/pep-0008/#tabs-or-spaces)
while문
- 조건이 true인 경우 명령이 계속 실행되는 반복문이고, 조건이 거짓이 될 때 while 반복문을 빠져나옴.
while something_is_true:
# Do this
# Then do this
# Then do this
def turn_right():
turn_left()
turn_left()
turn_left()
def jump():
move()
turn_left()
move()
turn_right()
move()
turn_right()
move()
turn_left()
number_of_hurdles = 6
while number_of_hurdles > 0:
jump()
number_of_hurdles -= 1
print(number_of_hurdles)
- 위 로봇이 블록을 넘는 게임의 코드를 for문이 아닌 while문으로 다시 작성함.
- while에서는 number_of_hurdles가 0이 되면 0은 0보다 크지 않기 때문에 false가 됨.
❓ 로봇이 움직이게 하는 게임 과제 실행하기
Q1. 블록 어딘가에 깃발이 있으며, at_goal()에 도달할 때 끝나는 코드를 생성
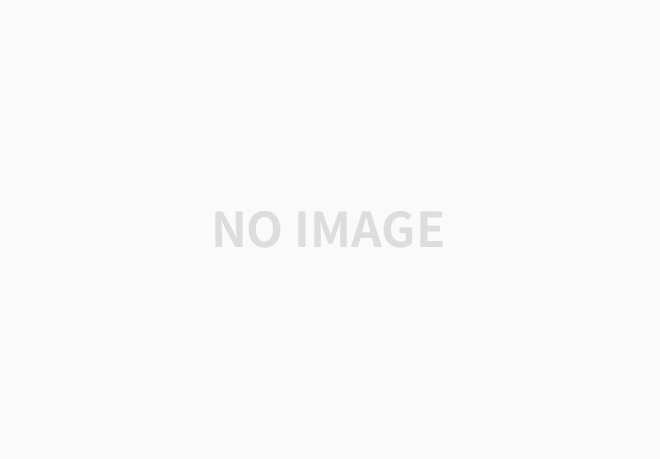
def turn_right():
turn_left()
turn_left()
turn_left()
def jump():
move()
turn_left()
move()
turn_right()
move()
turn_right()
move()
turn_left()
while at_goal() != True:
jump()
while not at_goal():
jump()
Q1. 블록 어딘가에 깃발이 있으며, at_goal()에 도달할 때 끝나는데 블록 형태가 매번 바뀌어도 통과할 수 있어야함.
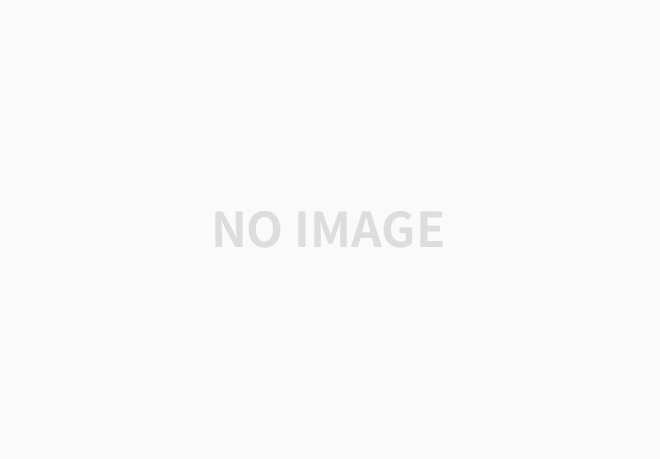
def turn_right():
turn_left()
turn_left()
turn_left()
def jump():
move()
turn_left()
move()
turn_right()
move()
turn_right()
move()
turn_left()
def wall_front():
turn_left()
move()
turn_right()
move()
turn_right()
move()
turn_left()
while not at_goal():
if front_is_clear() != True:
wall_front()
else:
move()
→ jump() 함수를 수정해서 쓰면 됐는데, 수정할 생각 안하고 새로 만들어서 사용함.
if 조건문을 앞에 벽이 있다면인데, 가독성 없게 코드 만든듯 (if wall_in_front(): 로 사용할 수 있음)
그리고 벽이 없을 경우 move()하는 것으로 코드 만듦.
Q. 블록 어딘가에 깃발이 있으며, at_goal()에 도달할 때 끝나는데 블록 높이와 형태가 매번 바뀌어도 통과할 수 있어야함.
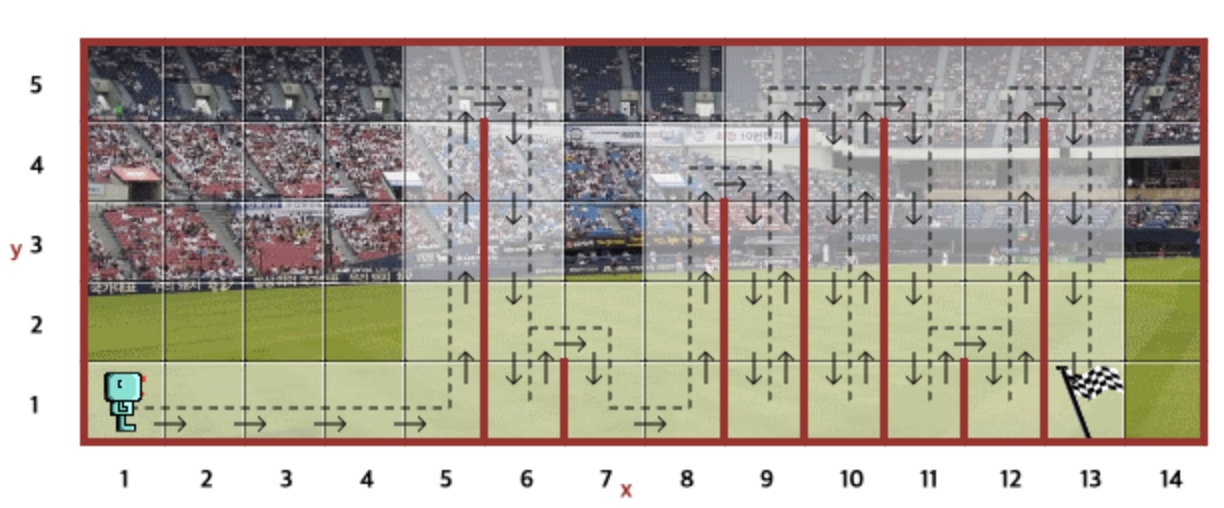
def turn_right():
turn_left()
turn_left()
turn_left()
def jump():
turn_left()
while wall_on_right():
move()
turn_right()
move()
turn_right()
while front_is_clear():
move()
turn_left()
while not at_goal():
if wall_in_front():
jump()
else:
move()
6일차 프로젝트
미로 탈출
- 로봇의 위치는 매번 달라지며, goal 지점으로 갈 수 있게 코드 생성
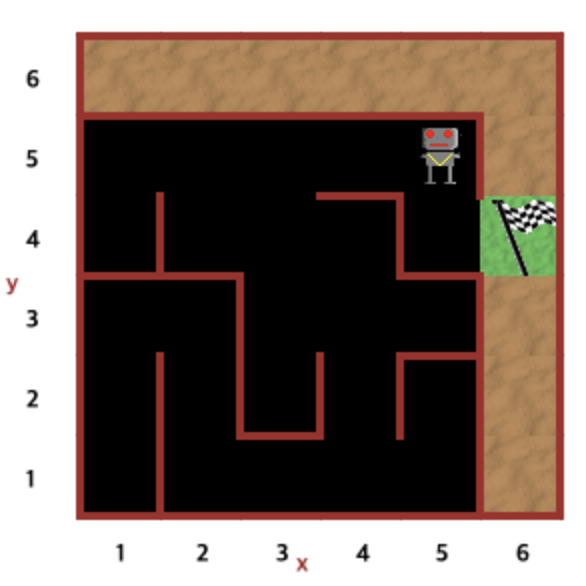
Reeborg was exploring a dark maze and the battery in its flashlight ran out.
Write a program using an if/elif/else statement so Reeborg can find the exit. The secret is to have Reeborg follow along the right edge of the maze, turning right if it can, going straight ahead if it can’t turn right, or turning left as a last resort.
- The functions move() and turn_left().
- Either the test front_is_clear() or wall_in_front(), right_is_clear() or wall_on_right(), and at_goal().
- How to use a while loop and if/elif/else statements.
- It might be useful to know how to use the negation of a test (not in Python).
def turn_right():
turn_left()
turn_left()
turn_left()
while not at_goal():
if right_is_clear():
turn_right()
move()
elif front_is_clear():
move()
else:
turn_left()
p.s 난 바보인가
'Language > Python' 카테고리의 다른 글
[Section8] 함수의 매개변수와 카이사르 암호 (1) | 2025.01.16 |
---|---|
[Section7] 게임 프로젝트(행맨) (0) | 2025.01.14 |
[Section5] 파이썬 반복문 (0) | 2025.01.13 |
[Section4] 파이썬 리스트 (1) | 2025.01.12 |
[Section3] 흐름 제어와 논리 연산자 (0) | 2025.01.10 |